ConstraintCommons.jl
ConstraintCommons.jl is an essential package within the Julia Constraints ecosystem designed to facilitate the development and interoperability of constraint programming solutions in Julia. It serves as a foundational layer that provides shared structures, abstract types, functions, and generic methods utilized by both basic feature packages and learning-oriented packages.
Only advanced users or package developers are likely to use it. The package covers parameters, (regular) languages, Core
or Base
methods extensions, sampling, extrema, and dictionaries.
Parameters
This section of the package list or extract parameters based on the XCSP3-core specifications. Note that, for the foreseeable future, the default constraints specification will follow these specifications.
ConstraintCommons.USUAL_CONSTRAINT_PARAMETERS Constant
const USUAL_CONSTRAINT_PARAMETERS
List of usual constraints parameters (based on XCSP3-core
constraints). The list is based on the nature of each kind of parameter instead of the keywords used in the XCSP3-core
format.
const USUAL_CONSTRAINT_PARAMETERS = [
:bool, # boolean parameter
:dim, # dimension, an integer parameter used along the pair_vars or vals parameters
:id, # index to target one variable in the input vector
:language, # describe a regular language such as an automaton or a MDD
:op, # an operator such as comparison or arithmetic operator
:pair_vars, # a list of parameters that are paired with each variable in the input vector
:val, # one scalar value
:vals, # a list of scalar values (independent of the input vector size)
]
ConstraintCommons.extract_parameters Function
extract_parameters(m::Union{Method, Function}; parameters)
Extracts the intersection between the kargs
of m
and parameters
(defaults to USUAL_CONSTRAINT_PARAMETERS
).
extract_parameters(s::Symbol, constraints_dict=USUAL_CONSTRAINTS; parameters=ConstraintCommons.USUAL_CONSTRAINT_PARAMETERS)
Return the parameters of the constraint s
in constraints_dict
.
Arguments
s::Symbol
: the constraint name.constraints_dict::Dict{Symbol,Constraint}
: dictionary of constraints. Default isUSUAL_CONSTRAINTS
.parameters::Vector{Symbol}
: vector of parameters. Default isConstraintCommons.USUAL_CONSTRAINT_PARAMETERS
.
Example
extract_parameters(:all_different)
Performances
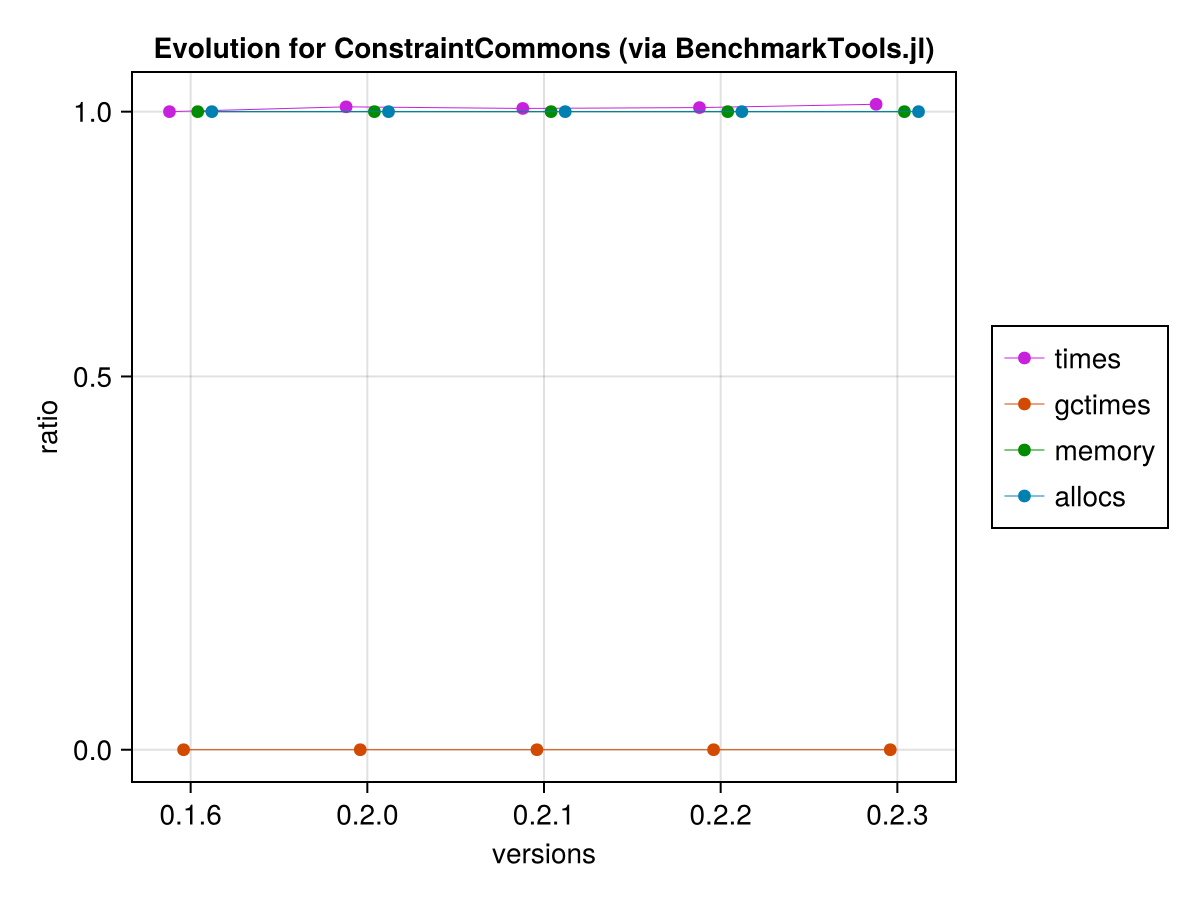
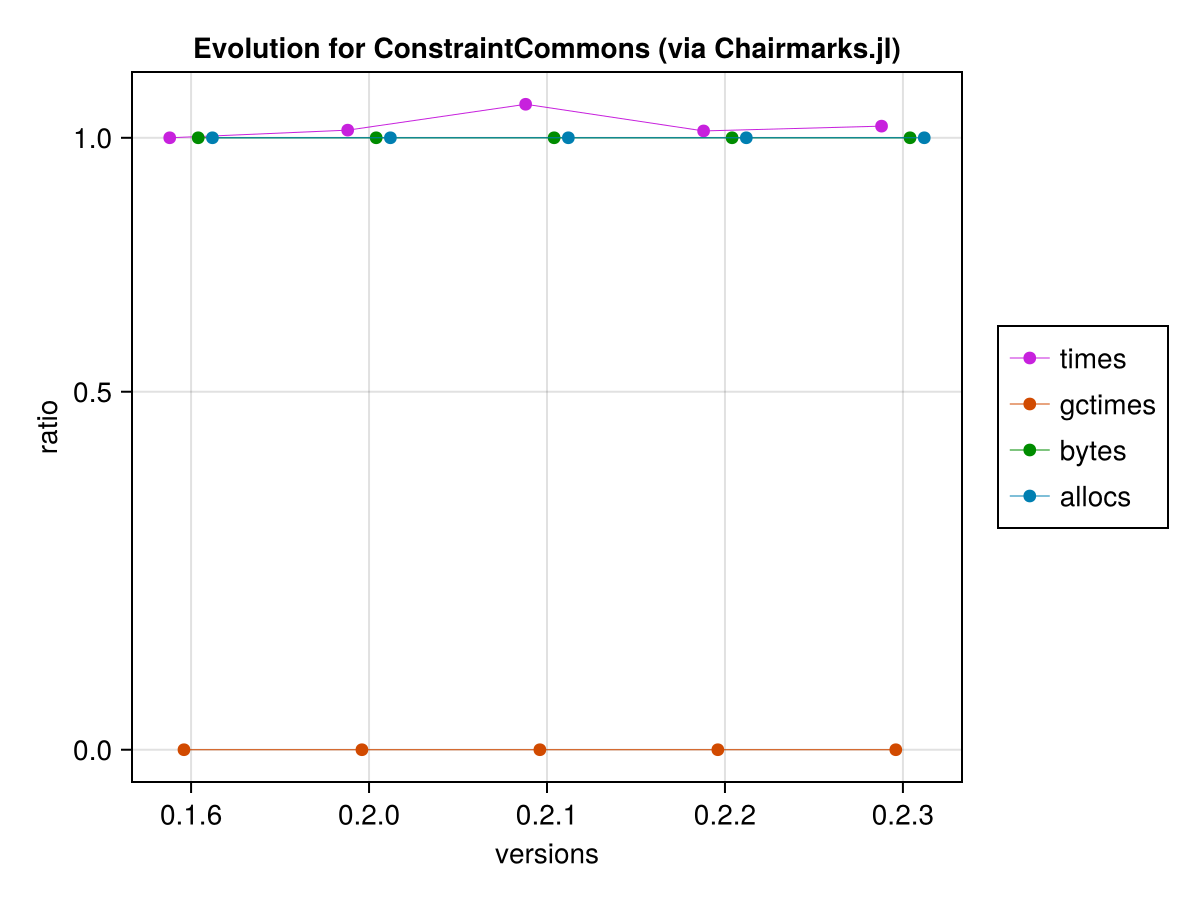
Languages
XCSP3 considers two kinds of structure to recognize languages as core constraints: Automata, Multivalued Decision Diagrams (MMDs).
ConstraintCommons.AbstractMultivaluedDecisionDiagram Type
AbstractMultivaluedDecisionDiagram
An abstract interface for Multivalued Decision Diagrams (MDD) used in Julia Constraints packages. Requirements:
accept(a<:AbstractMultivaluedDecisionDiagram, word)
: returntrue
ifa
acceptsword
.
ConstraintCommons.MDD Type
MDD{S,T} <: AbstractMultivaluedDecisionDiagram
A minimal implementation of a multivalued decision diagram structure.
ConstraintCommons.AbstractAutomaton Type
AbstractAutomaton
An abstract interface for automata used in Julia Constraints packages. Requirements:
accept(a<:AbstractAutomaton, word)
: returntrue
ifa
acceptsword
.
ConstraintCommons.Automaton Type
Automaton{S, T, F <: Union{S, Vector{S}, Set{S}}} <: AbstractAutomaton
A minimal implementation of a deterministic automaton structure.
ConstraintCommons.accept Function
accept(a::Union{Automaton, MDD}, w)
Return true
if a
accepts the word w
and false
otherwise.
ConstraintCommons.accept(fa::FakeAutomaton, word)
Implement the accept
methods for FakeAutomaton
.
ConstraintCommons.at_end Function
at_end(a::Automaton, s)
Internal method used by accept
with Automaton
.
Performances
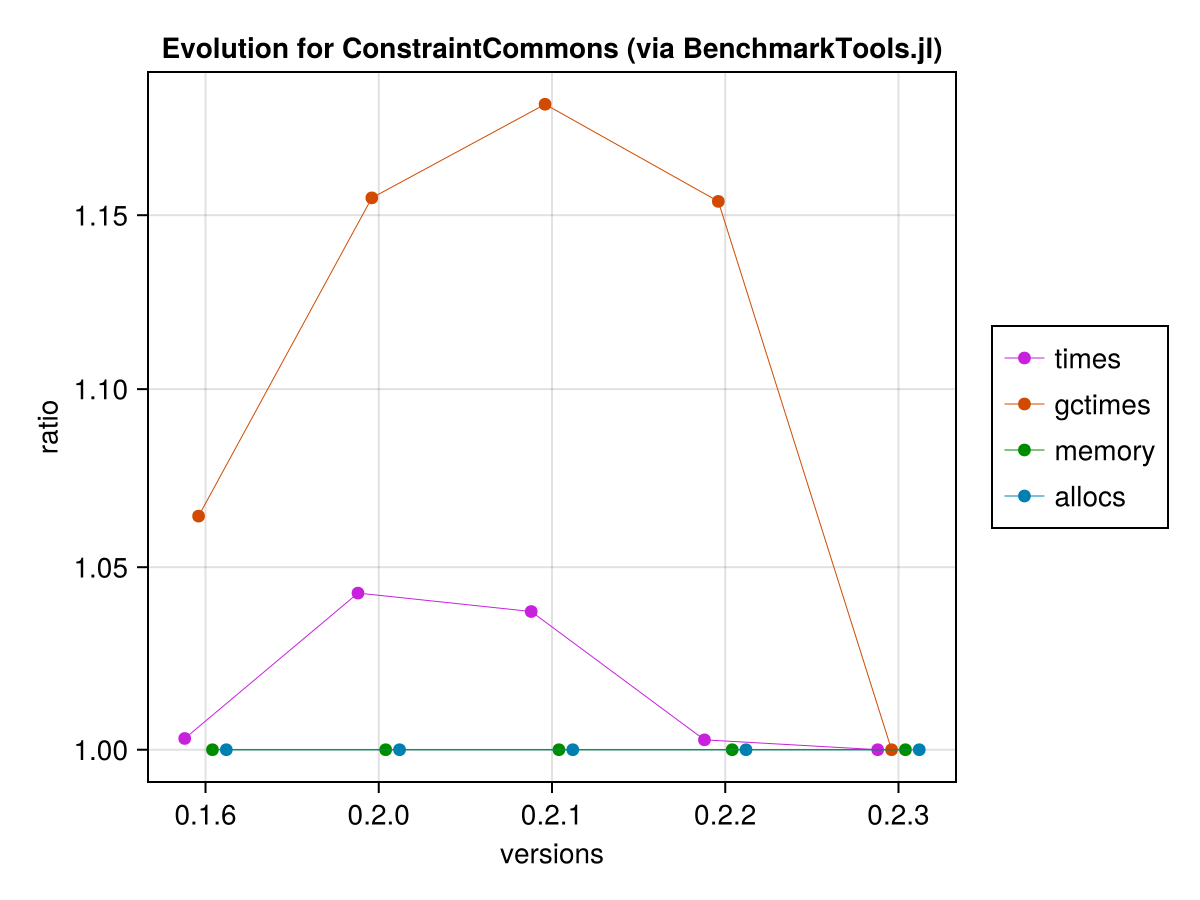
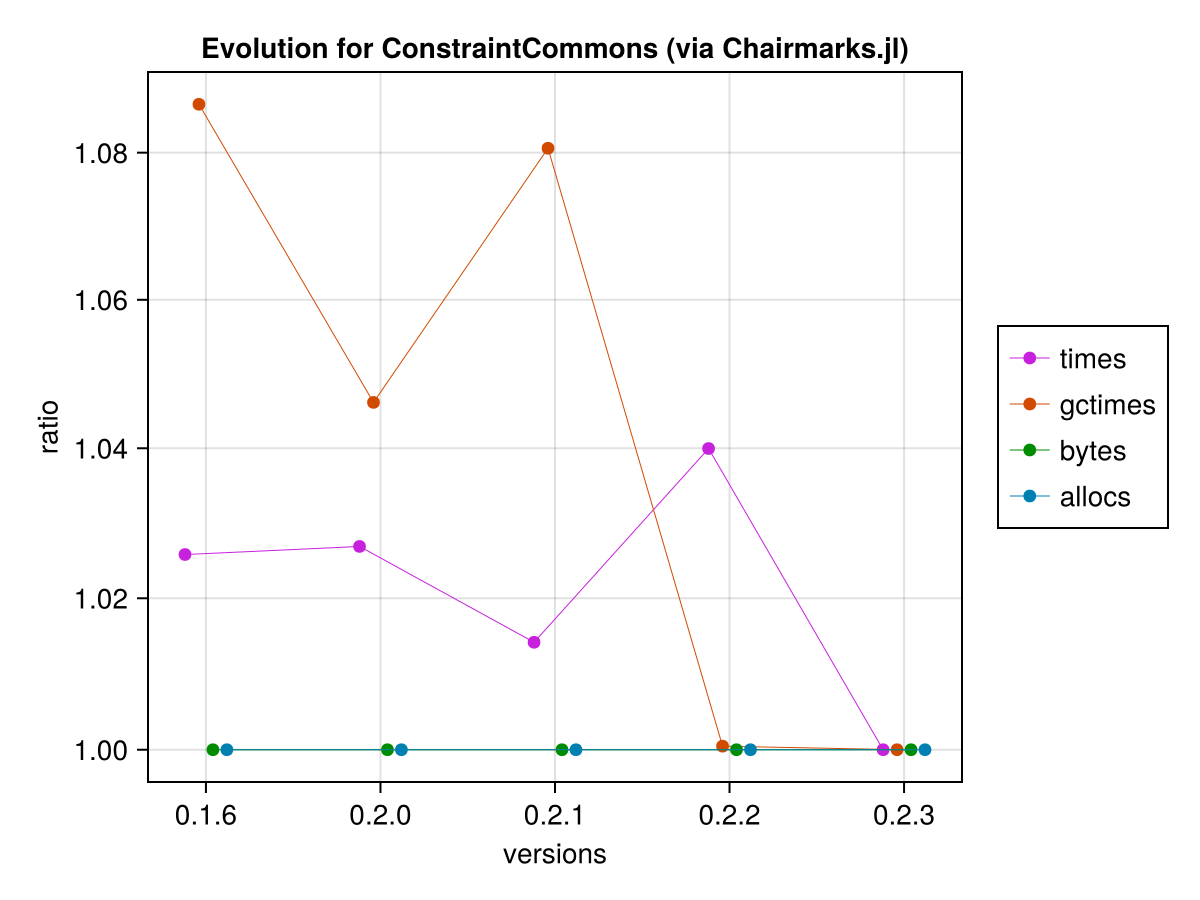
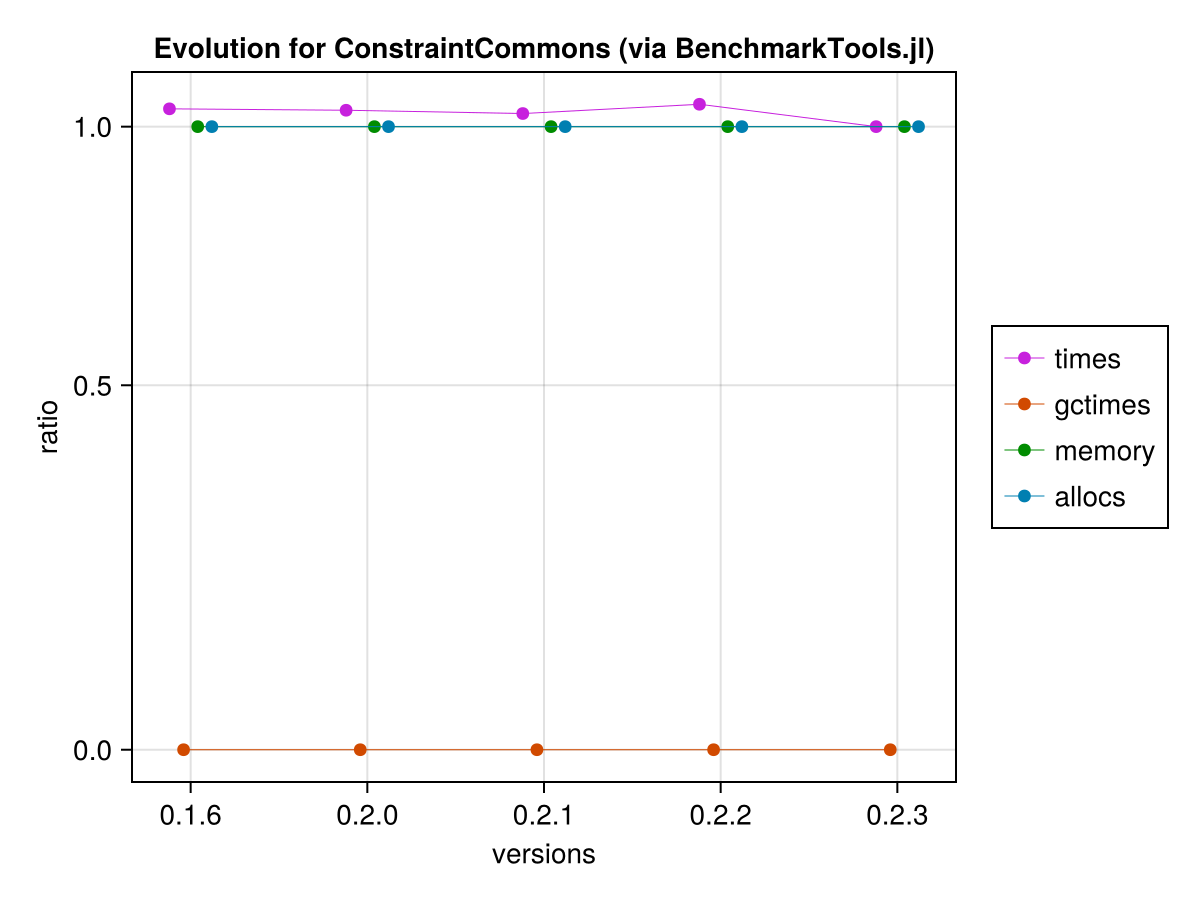
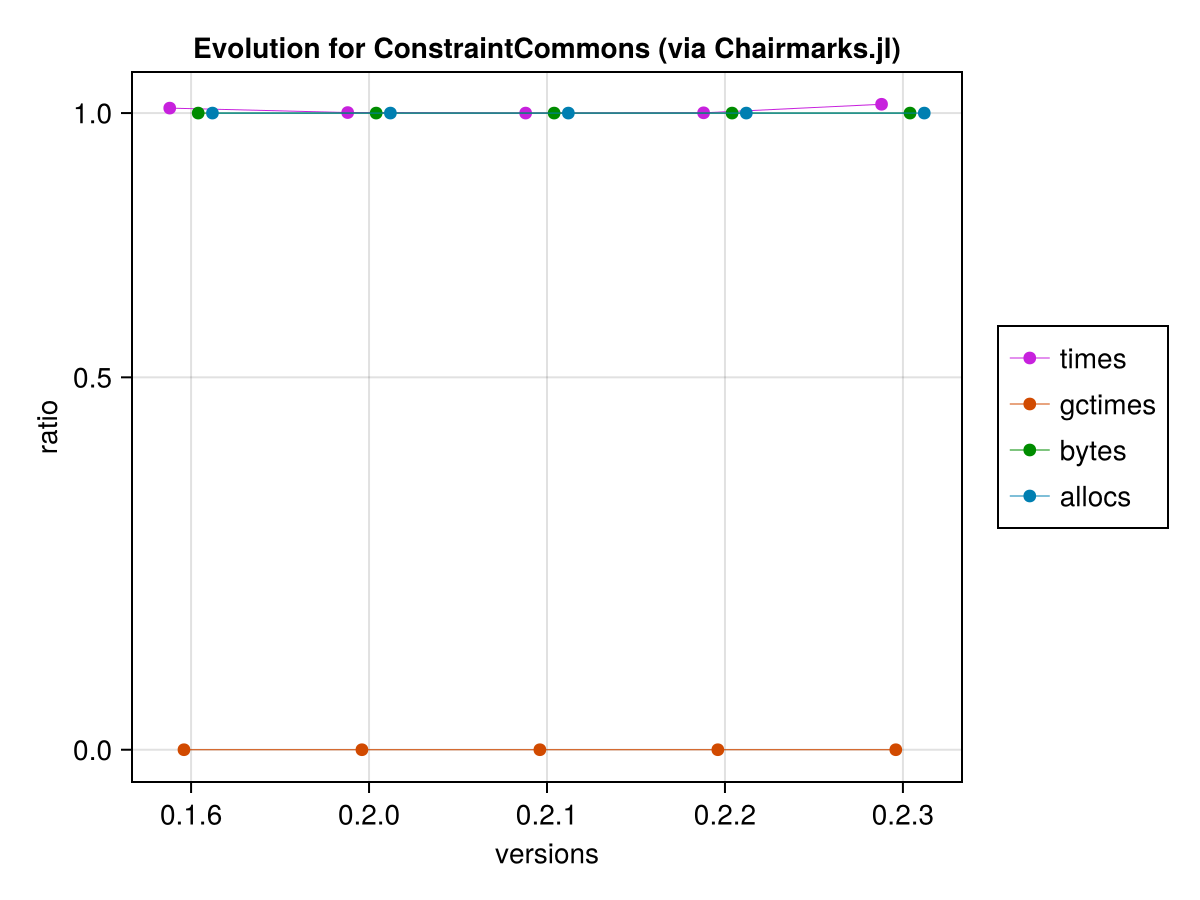
Extensions
We extend some operations for Nothing
and Symbol
.
ConstraintCommons.symcon Function
symcon(s1::Symbol, s2::Symbol, connector::AbstractString="_")
Extends *
to Symbol
s multiplication by connecting the symbols by an _
.
ConstraintCommons.consin Function
consin(::Any, ::Nothing)
Extends Base.in
(or ∈
) when the set is nothing
. Returns false
.
ConstraintCommons.consisempty Function
consisempty(::Nothing)
Extends Base.isempty
when the set is nothing
. Returns true
.
Performances
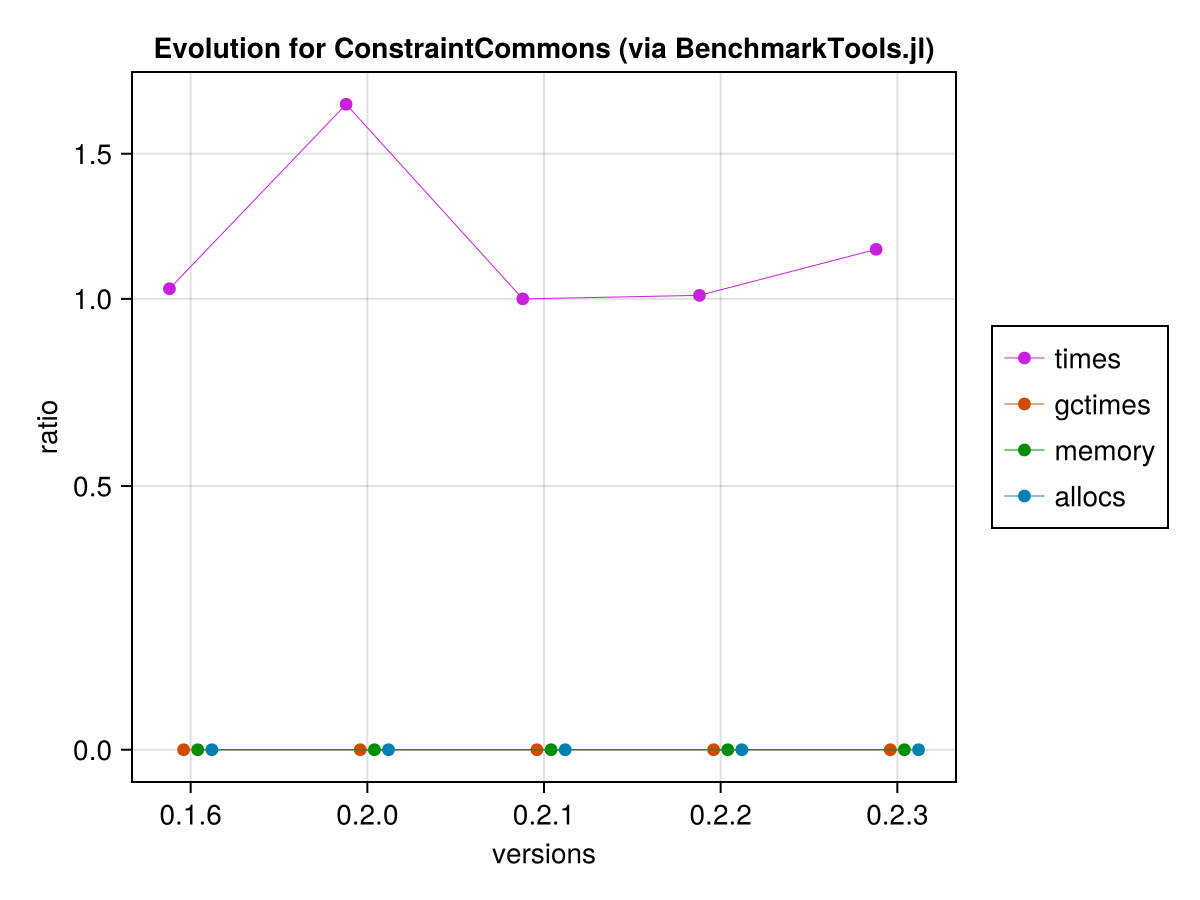
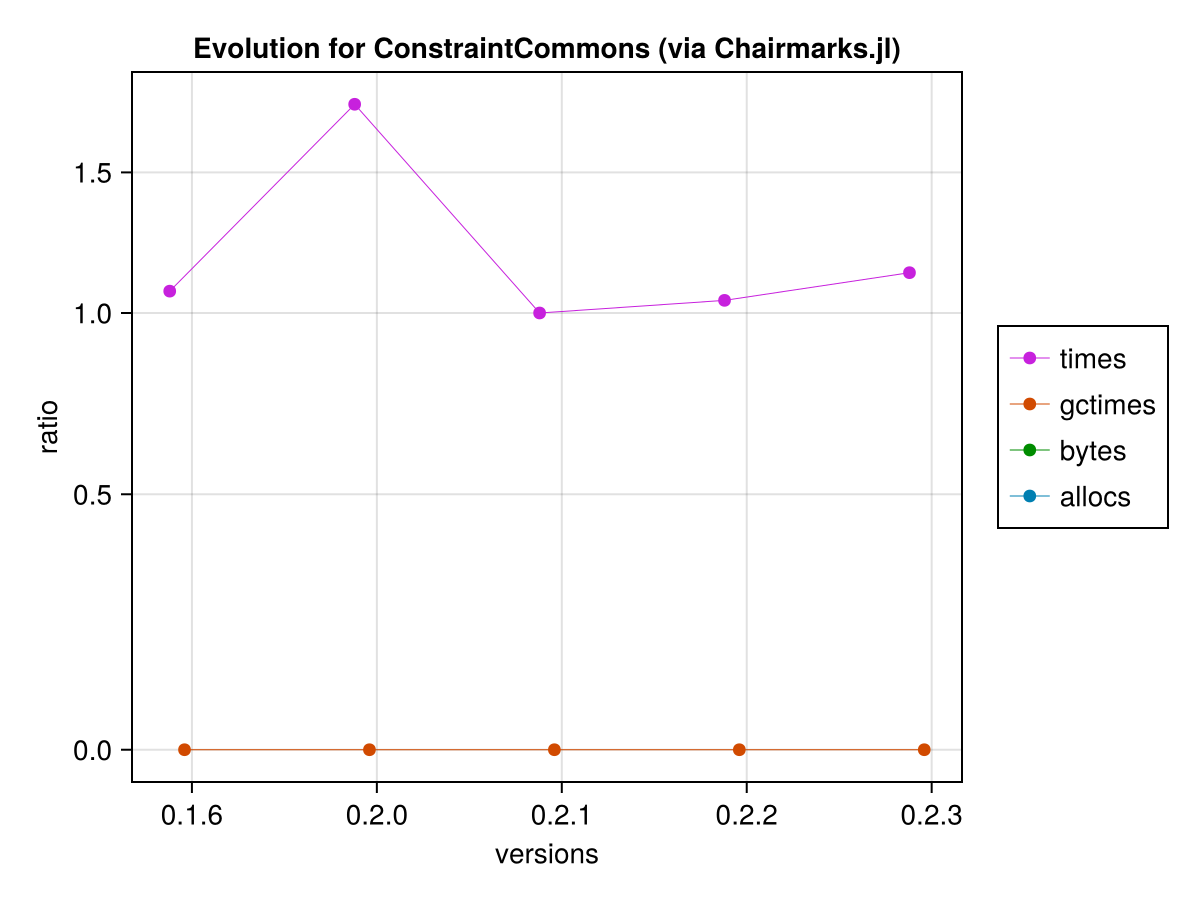
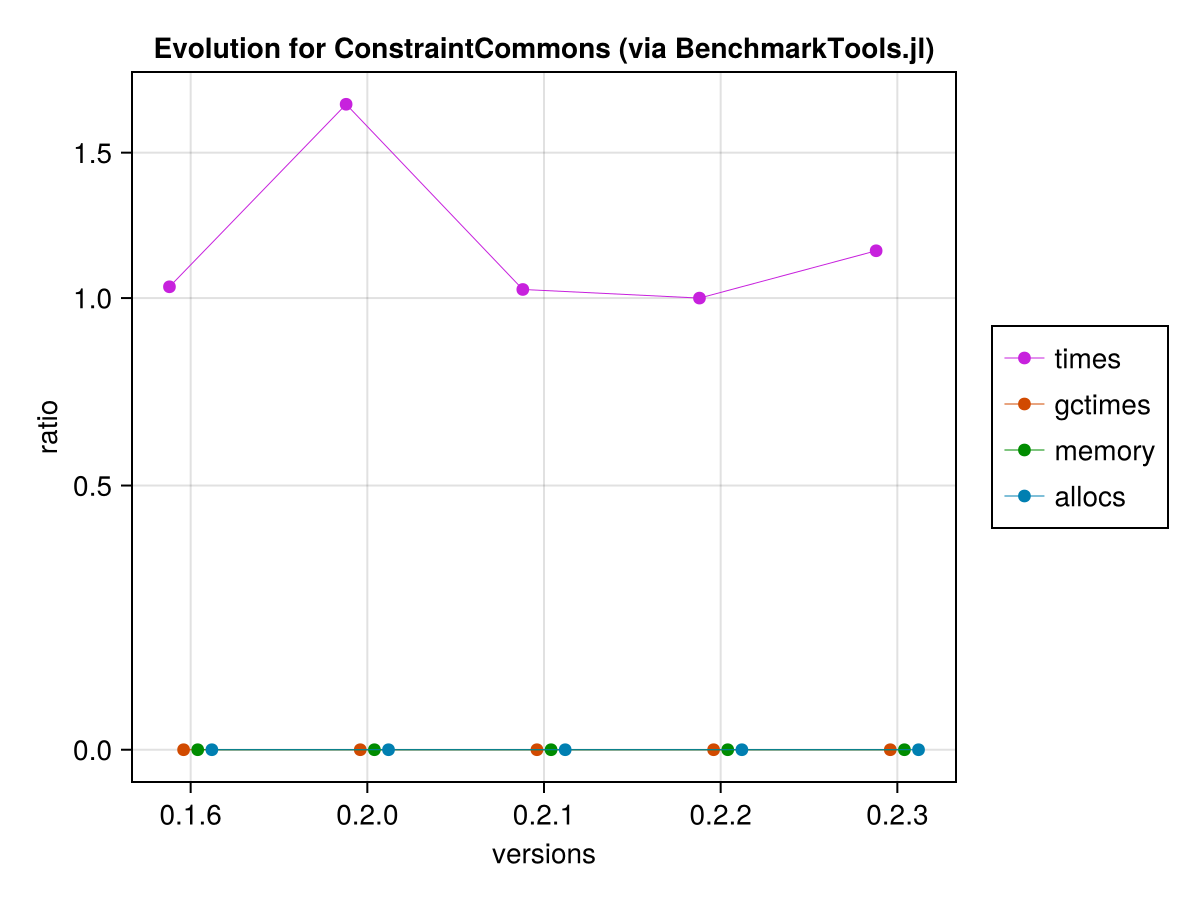
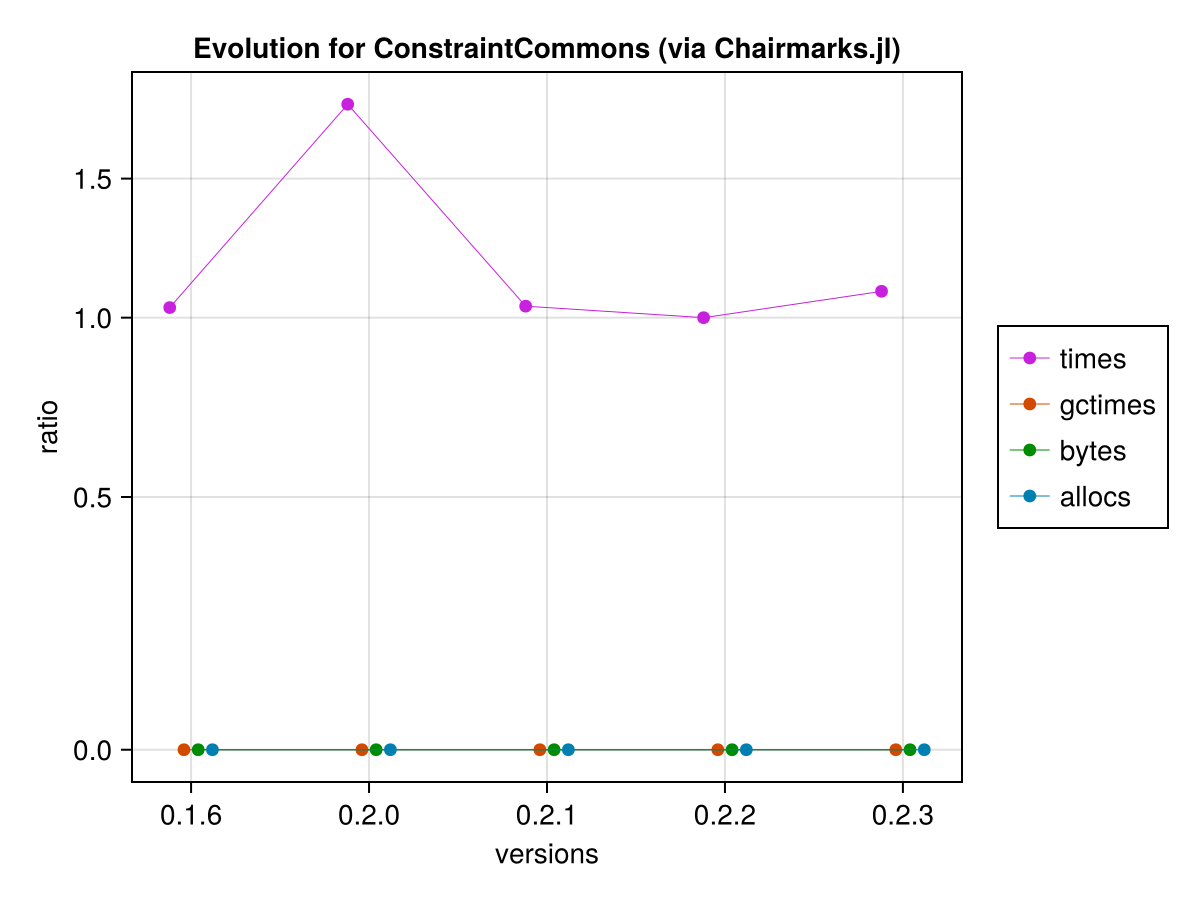
Sampling
During our constraint learning processes, we use sampling to efficiently make partial exploration of search spaces. The following are some examples of sampling utilities.
ConstraintCommons.oversample Function
oversample(X, f)
Oversample elements of X
until the boolean function f
has as many true
and false
configurations.
Performances


Extrema
We need to compute the difference between extrema of various kind of collections in several situations.
ConstraintCommons.δ_extrema Function
δ_extrema(X...)
Compute both the difference between the maximum and the minimum of over all the collections of X
.
Performances
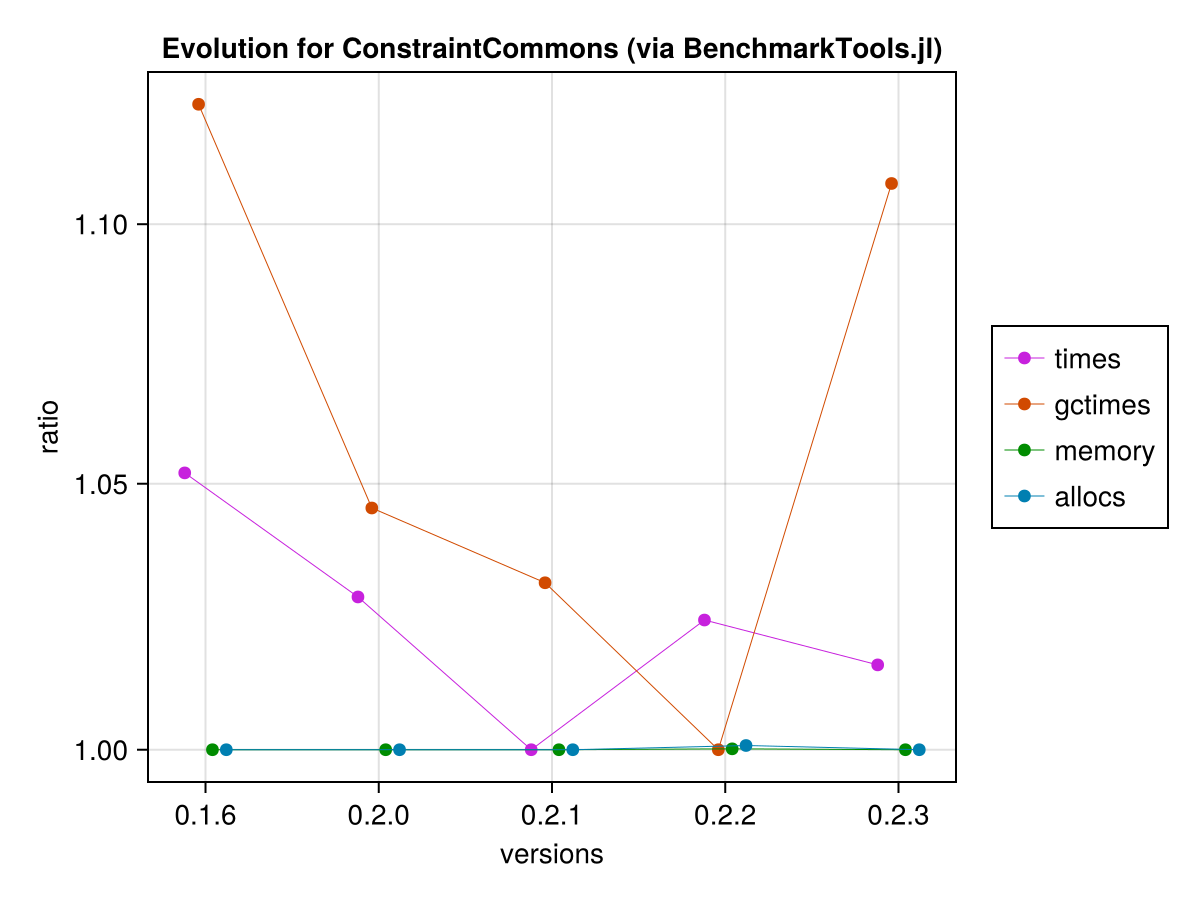
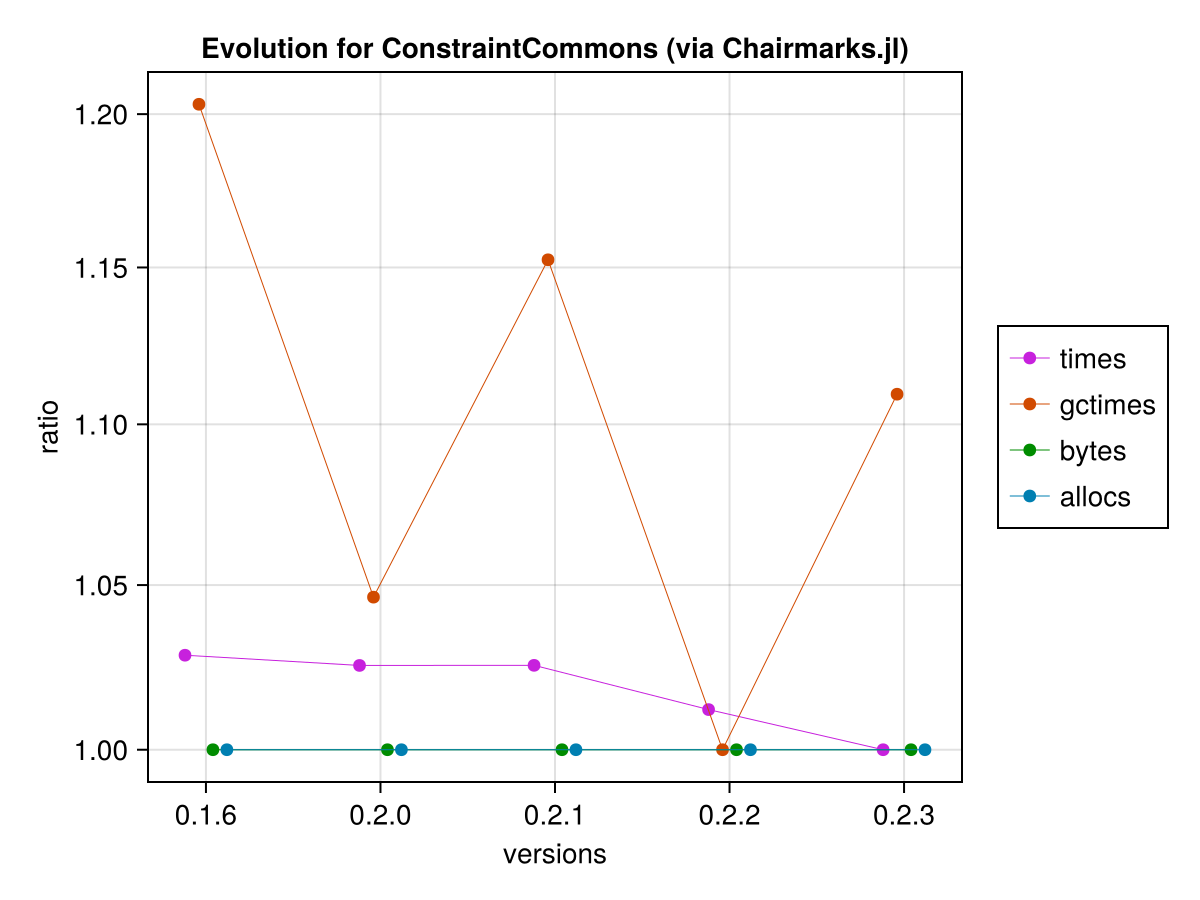
Dictionaries
We provide the ever-useful incsert!
function for dictionaries.
ConstraintCommons.incsert! Function
incsert!(d::Union{AbstractDict, AbstractDictionary}, ind, val = 1)
Increase or insert a counter in a dictionary-based collection. The counter insertion defaults to val = 1
.
Performances
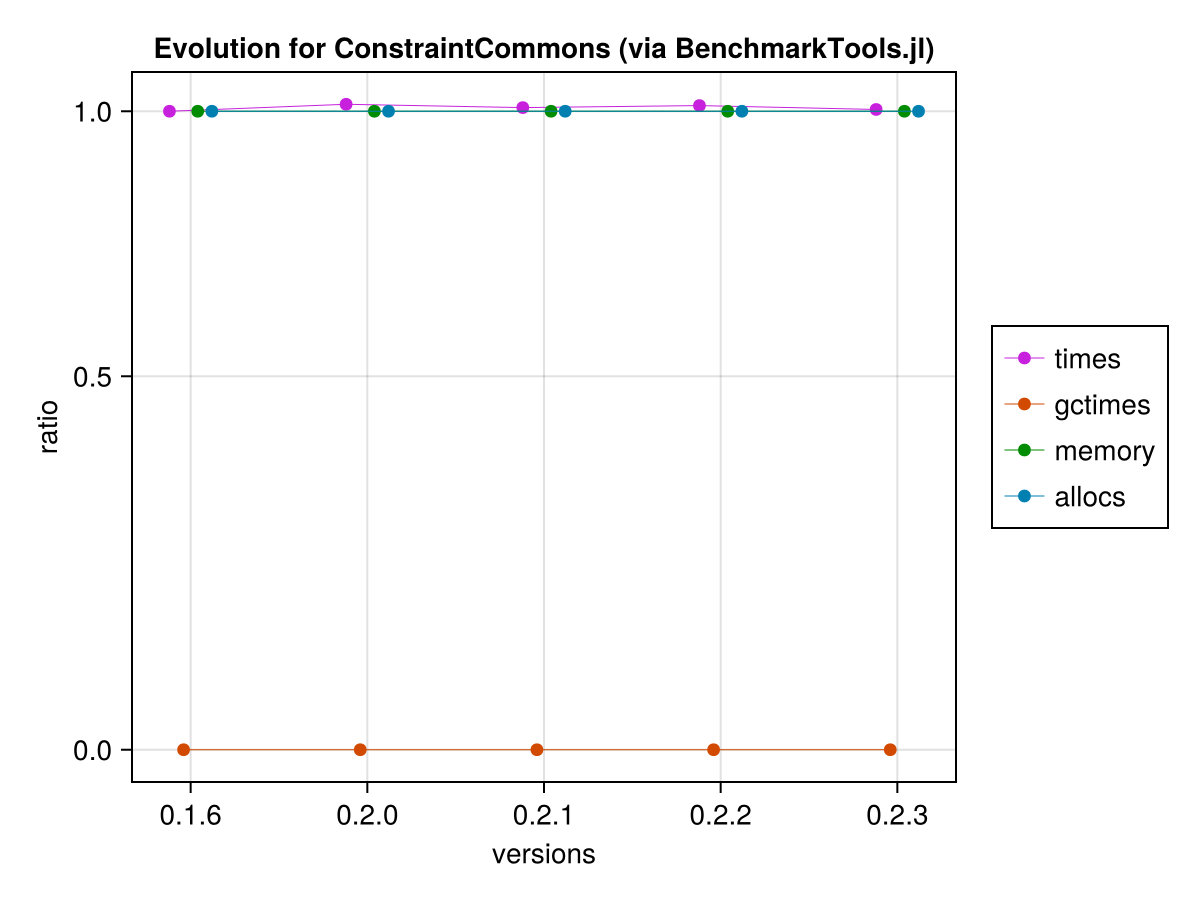
