ConstraintDomains.jl: Defining Variables and Exploring Domains
ConstraintDomains.jl is the standard way to define variables and explore domains in the Julia Constraints ecosystem. This package can be used to specify both discrete and continuous domains. Explorations features are primarily related to learning about constraints, aka constraint learning.
Most users should use it through JuMP/MOI interfaces.
Commons
ConstraintDomains.AbstractDomain Type
AbstractDomain
An abstract super type for any domain type. A domain type D <: AbstractDomain
must implement the following methods to properly interface AbstractDomain
.
Base.∈(val, ::D)
Base.rand(::D)
Base.length(::D)
that is the number of elements in a discrete domain, and the distance between bounds or similar for a continuous domain
Additionally, if the domain is used in a dynamic context, it can extend
add!(::D, args)
delete!(::D, args)
where args
depends on D
's structure
ConstraintDomains.domain Function
domain()
Construct an EmptyDomain
.
domain(a::Tuple{T, Bool}, b::Tuple{T, Bool}) where {T <: Real}
domain(intervals::Vector{Tuple{Tuple{T, Bool},Tuple{T, Bool}}}) where {T <: Real}
Construct a domain of continuous interval(s).
domain(values)
domain(range::R) where {T <: Real, R <: AbstractRange{T}}
Construct either a SetDomain
or a RangeDomain
.
d1 = domain(1:5)
d2 = domain([53.69, 89.2, 0.12])
d3 = domain([2//3, 89//123])
d4 = domain(4.3)
d5 = domain(1,42,86.9)
ConstraintDomains.domain_size Function
domain_size(d <: AbstractDomain)
Fallback method for domain_size(d)
that return length(d)
.
domain_size(itv::Intervals)
Return the difference between the highest and lowest values in itv
.
domain_size(d::D) where D <: DiscreteDomain
Return the maximum distance between two points in d
.
ConstraintDomains.get_domain Function
get_domain(::AbstractDomain)
Access the internal structure of any domain type.
ConstraintDomains.to_domains Function
to_domains(args...)
Convert various arguments into valid domains format.
Extension to Base module
Base.in Function
x::Variable ∈ constraint
value ∈ x::Variable
Check if a variable x
is restricted by a constraint::Int
, or if a value
belongs to the domain of x
.
var::Int ∈ c::Constraint
Base.in(value, d <: AbstractDomain)
Fallback method for value ∈ d
that returns false
.
Base.in(x, itv::Intervals)
Return true
if x ∈ I
for any 'I ∈ itv, false otherwise.
x ∈ I` is equivalent to
a < x < b
ifI = (a, b)
a < x ≤ b
ifI = (a, b]
a ≤ x < b
ifI = [a, b)
a ≤ x ≤ b
ifI = [a, b]
Base.in(value, d::D) where D <: DiscreteDomain
Return true
if value
is a point of d
.
Base.rand Function
Base.rand(::Vector{IntervalsFold})
Extend the Base.rand
function to Vector{IntervalsFold}
.
rand(pf<:PatternFold)
Returns a random value of pf
as if it was unfolded.
Base.rand(::Vector{AbstractVectorFold})
Extend the `Base.rand` function to `Vector{AbstractVectorFold}`.
Base.rand(d::Union{Vector{D},Set{D}, D}) where {D<:AbstractDomain}
Extends Base.rand
to (a collection of) domains.
Base.rand(itv::Intervals)
Base.rand(itv::Intervals, i)
Return a random value from itv
, specifically from the i
th interval if i
is specified.
Base.rand(d::D) where D <: DiscreteDomain
Draw randomly a point in d
.
Base.rand(fa::FakeAutomaton)
Extends Base.rand
. Currently simply returns fa
.
Base.isempty Function
Base.isempty(d <: AbstractDomain)
Fallback method for isempty(d)
that return length(d) == 0
which default to 0
.
Base.rand Function
Base.rand(::Vector{IntervalsFold})
Extend the Base.rand
function to Vector{IntervalsFold}
.
rand(pf<:PatternFold)
Returns a random value of pf
as if it was unfolded.
Base.rand(::Vector{AbstractVectorFold})
Extend the `Base.rand` function to `Vector{AbstractVectorFold}`.
Base.rand(d::Union{Vector{D},Set{D}, D}) where {D<:AbstractDomain}
Extends Base.rand
to (a collection of) domains.
Base.rand(itv::Intervals)
Base.rand(itv::Intervals, i)
Return a random value from itv
, specifically from the i
th interval if i
is specified.
Base.rand(d::D) where D <: DiscreteDomain
Draw randomly a point in d
.
Base.rand(fa::FakeAutomaton)
Extends Base.rand
. Currently simply returns fa
.
Base.string Function
Base.string(D::Vector{<:AbstractDomain})
Base.string(d<:AbstractDomain)
Extends the string
method to (a vector of) domains.
Performances

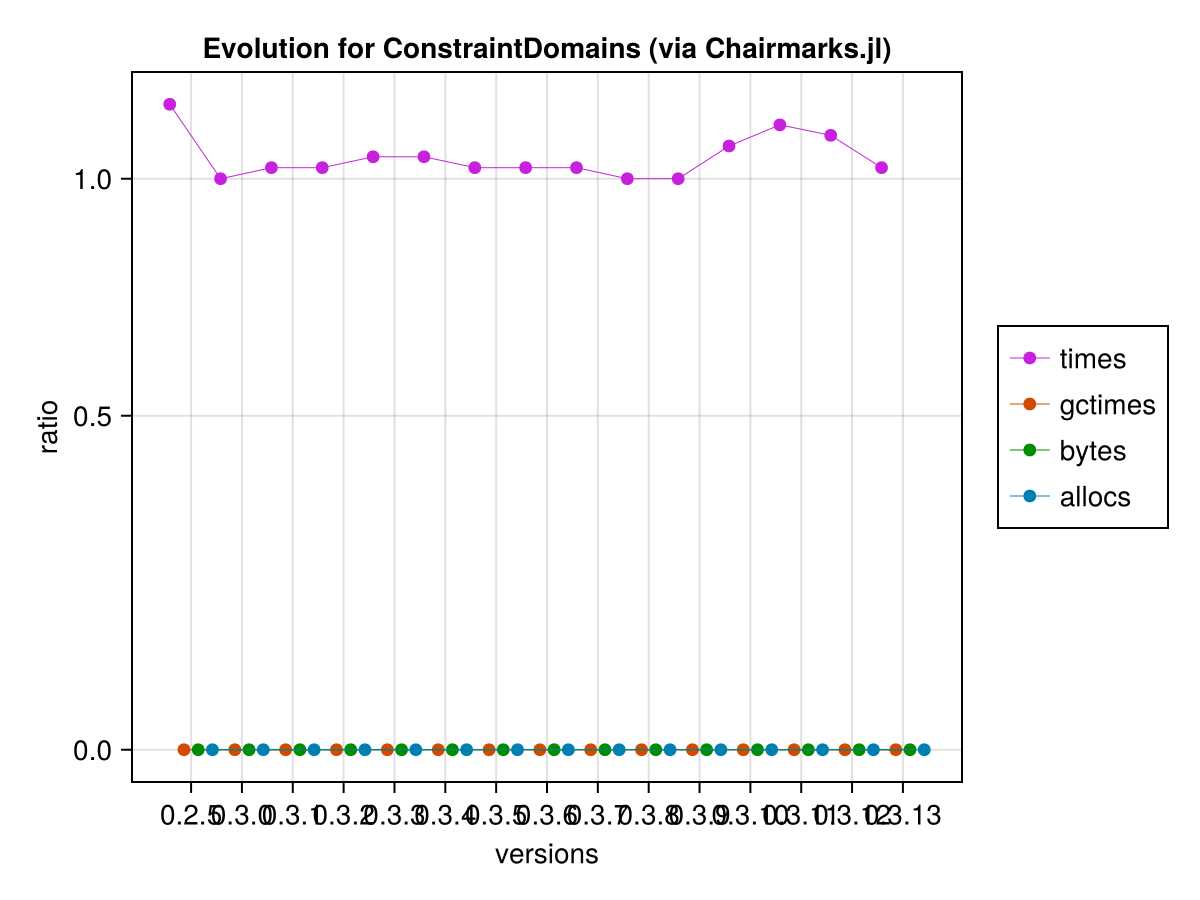
Continuous
ConstraintDomains.ContinuousDomain Type
ContinuousDomain{T <: Real} <: AbstractDomain
An abstract supertype for all continuous domains.
ConstraintDomains.Intervals Type
Intervals{T <: Real} <: ContinuousDomain{T}
An encapsuler to store a vector of PatternFolds.Interval
. Dynamic changes to Intervals
are not handled yet.
ConstraintDomains.domain Function
domain()
Construct an EmptyDomain
.
domain(a::Tuple{T, Bool}, b::Tuple{T, Bool}) where {T <: Real}
domain(intervals::Vector{Tuple{Tuple{T, Bool},Tuple{T, Bool}}}) where {T <: Real}
Construct a domain of continuous interval(s).
domain(values)
domain(range::R) where {T <: Real, R <: AbstractRange{T}}
Construct either a SetDomain
or a RangeDomain
.
d1 = domain(1:5)
d2 = domain([53.69, 89.2, 0.12])
d3 = domain([2//3, 89//123])
d4 = domain(4.3)
d5 = domain(1,42,86.9)
ConstraintDomains.domain_size Function
domain_size(d <: AbstractDomain)
Fallback method for domain_size(d)
that return length(d)
.
domain_size(itv::Intervals)
Return the difference between the highest and lowest values in itv
.
domain_size(d::D) where D <: DiscreteDomain
Return the maximum distance between two points in d
.
ConstraintDomains.merge_domains Function
merge_domains(d₁::AbstractDomain, d₂::AbstractDomain)
Merge two domains of same nature (discrete/contiuous).
ConstraintDomains.intersect_domains Function
intersect_domains(d₁, d₂)
Compute the intersections of two domains.
ConstraintDomains.intersect_domains! Function
intersect_domains!(is, i, new_itvls)
Compute the intersections of a domain with an interval and store the results in new_itvls
.
Arguments
is::IS
: a collection of intervals.i::I
: an interval.new_itvls::Vector{I}
: a vector to store the results.
ConstraintDomains.size Function
Base.size(i::I) where {I <: Interval}
Defines the size of an interval as its span
.
Extension to Base module
Base.length Function
length(layer)
Return the number of operations in a layer.
Base.length(icn)
Return the total number of operations of an ICN.
length(pf<:PatternFold)
Return the length of pf
if unfolded.
Base.rand(d <: AbstractDomain)
Fallback method for length(d)
that return 0
.
Base.length(itv::Intervals)
Return the sum of the length of each interval in itv
.
Base.length(d::D) where D <: DiscreteDomain
Return the number of points in d
.
Base.rand Function
Base.rand(::Vector{IntervalsFold})
Extend the Base.rand
function to Vector{IntervalsFold}
.
rand(pf<:PatternFold)
Returns a random value of pf
as if it was unfolded.
Base.rand(::Vector{AbstractVectorFold})
Extend the `Base.rand` function to `Vector{AbstractVectorFold}`.
Base.rand(d::Union{Vector{D},Set{D}, D}) where {D<:AbstractDomain}
Extends Base.rand
to (a collection of) domains.
Base.rand(itv::Intervals)
Base.rand(itv::Intervals, i)
Return a random value from itv
, specifically from the i
th interval if i
is specified.
Base.rand(d::D) where D <: DiscreteDomain
Draw randomly a point in d
.
Base.rand(fa::FakeAutomaton)
Extends Base.rand
. Currently simply returns fa
.
Base.in Function
x::Variable ∈ constraint
value ∈ x::Variable
Check if a variable x
is restricted by a constraint::Int
, or if a value
belongs to the domain of x
.
var::Int ∈ c::Constraint
Base.in(value, d <: AbstractDomain)
Fallback method for value ∈ d
that returns false
.
Base.in(x, itv::Intervals)
Return true
if x ∈ I
for any 'I ∈ itv, false otherwise.
x ∈ I` is equivalent to
a < x < b
ifI = (a, b)
a < x ≤ b
ifI = (a, b]
a ≤ x < b
ifI = [a, b)
a ≤ x ≤ b
ifI = [a, b]
Base.in(value, d::D) where D <: DiscreteDomain
Return true
if value
is a point of d
.
Base.string Function
Base.string(D::Vector{<:AbstractDomain})
Base.string(d<:AbstractDomain)
Extends the string
method to (a vector of) domains.
Performances
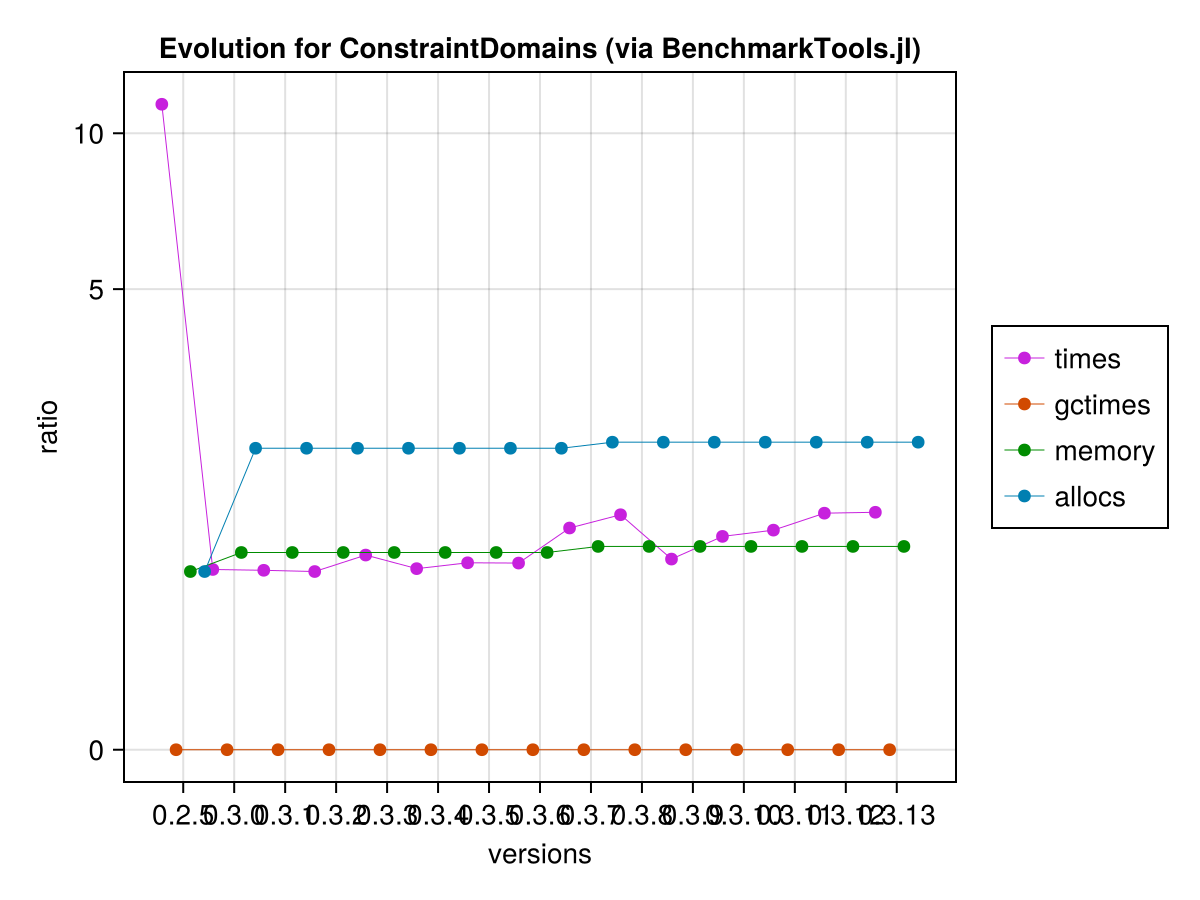
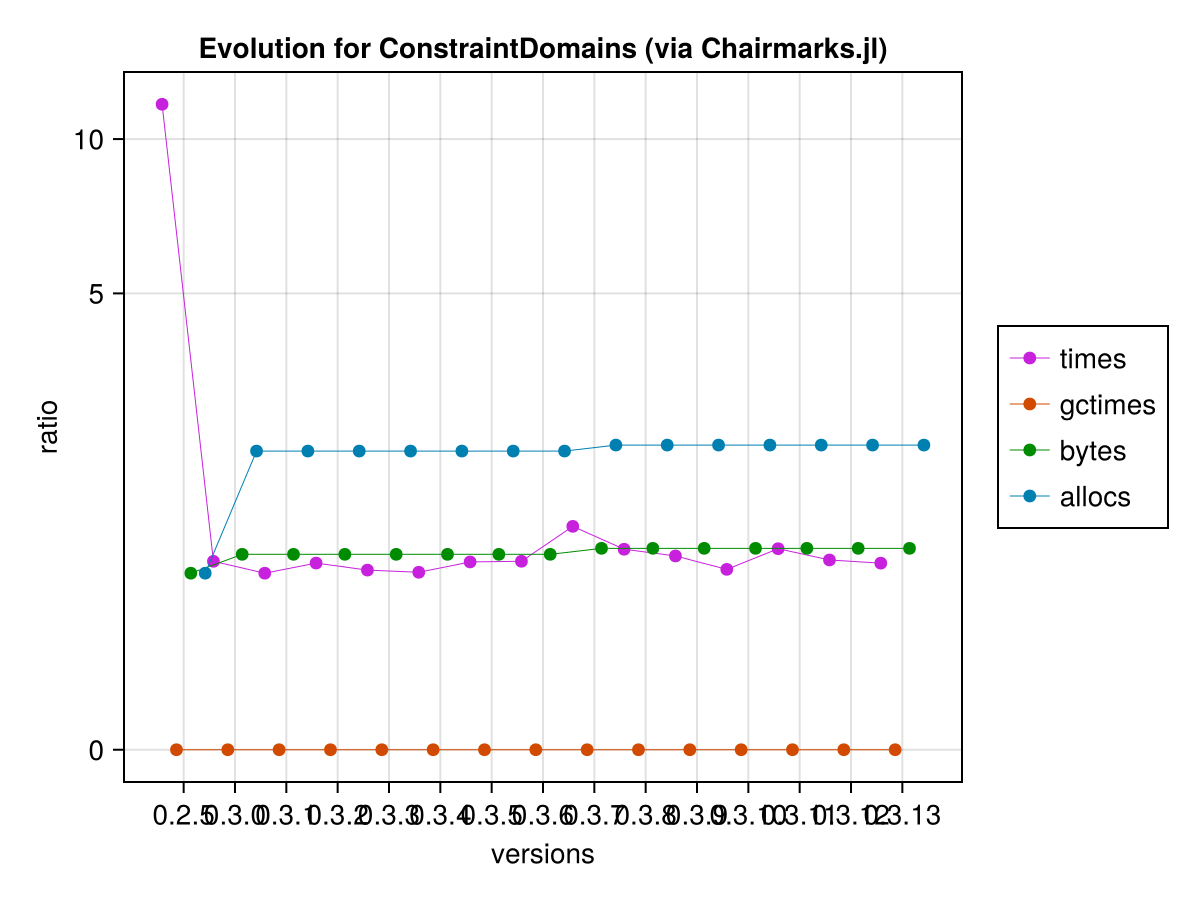
Discrete
ConstraintDomains.DiscreteDomain Type
DiscreteDomain{T <: Number} <: AbstractDomain
An abstract supertype for discrete domains (set, range).
ConstraintDomains.SetDomain Type
SetDomain{T <: Number} <: DiscreteDomain{T}
Domain that stores discrete values as a set of (unordered) points.
ConstraintDomains.RangeDomain Type
RangeDomain
A discrete domain defined by a range <: AbstractRange{Real}
. As ranges are immutable in Julia, changes in RangeDomain
must use set_domain!
.
ConstraintDomains.ArbitraryDomain Function
ArbitraryDomain{T} <: DiscreteDomain{T}
A domain type that stores arbitrary values, possibly non numeric, of type T
.
ConstraintDomains.domain Function
domain()
Construct an EmptyDomain
.
domain(a::Tuple{T, Bool}, b::Tuple{T, Bool}) where {T <: Real}
domain(intervals::Vector{Tuple{Tuple{T, Bool},Tuple{T, Bool}}}) where {T <: Real}
Construct a domain of continuous interval(s).
domain(values)
domain(range::R) where {T <: Real, R <: AbstractRange{T}}
Construct either a SetDomain
or a RangeDomain
.
d1 = domain(1:5)
d2 = domain([53.69, 89.2, 0.12])
d3 = domain([2//3, 89//123])
d4 = domain(4.3)
d5 = domain(1,42,86.9)
ConstraintDomains.domain_size Function
domain_size(d <: AbstractDomain)
Fallback method for domain_size(d)
that return length(d)
.
domain_size(itv::Intervals)
Return the difference between the highest and lowest values in itv
.
domain_size(d::D) where D <: DiscreteDomain
Return the maximum distance between two points in d
.
ConstraintDomains.merge_domains Function
merge_domains(d₁::AbstractDomain, d₂::AbstractDomain)
Merge two domains of same nature (discrete/contiuous).
ConstraintDomains.intersect_domains Function
intersect_domains(d₁, d₂)
Compute the intersections of two domains.
ConstraintDomains.size Function
Base.size(i::I) where {I <: Interval}
Defines the size of an interval as its span
.
Extension to Base module
Base.delete! Function
Base.delete!(d::SetDomain, value)(d::SetDomain, value)
Delete value
from the list of points in d
.
Base.length Function
length(layer)
Return the number of operations in a layer.
Base.length(icn)
Return the total number of operations of an ICN.
length(pf<:PatternFold)
Return the length of pf
if unfolded.
Base.rand(d <: AbstractDomain)
Fallback method for length(d)
that return 0
.
Base.length(itv::Intervals)
Return the sum of the length of each interval in itv
.
Base.length(d::D) where D <: DiscreteDomain
Return the number of points in d
.
Base.rand Function
Base.rand(::Vector{IntervalsFold})
Extend the Base.rand
function to Vector{IntervalsFold}
.
rand(pf<:PatternFold)
Returns a random value of pf
as if it was unfolded.
Base.rand(::Vector{AbstractVectorFold})
Extend the `Base.rand` function to `Vector{AbstractVectorFold}`.
Base.rand(d::Union{Vector{D},Set{D}, D}) where {D<:AbstractDomain}
Extends Base.rand
to (a collection of) domains.
Base.rand(itv::Intervals)
Base.rand(itv::Intervals, i)
Return a random value from itv
, specifically from the i
th interval if i
is specified.
Base.rand(d::D) where D <: DiscreteDomain
Draw randomly a point in d
.
Base.rand(fa::FakeAutomaton)
Extends Base.rand
. Currently simply returns fa
.
Base.in Function
x::Variable ∈ constraint
value ∈ x::Variable
Check if a variable x
is restricted by a constraint::Int
, or if a value
belongs to the domain of x
.
var::Int ∈ c::Constraint
Base.in(value, d <: AbstractDomain)
Fallback method for value ∈ d
that returns false
.
Base.in(x, itv::Intervals)
Return true
if x ∈ I
for any 'I ∈ itv, false otherwise.
x ∈ I` is equivalent to
a < x < b
ifI = (a, b)
a < x ≤ b
ifI = (a, b]
a ≤ x < b
ifI = [a, b)
a ≤ x ≤ b
ifI = [a, b]
Base.in(value, d::D) where D <: DiscreteDomain
Return true
if value
is a point of d
.
Base.string Function
Base.string(D::Vector{<:AbstractDomain})
Base.string(d<:AbstractDomain)
Extends the string
method to (a vector of) domains.
Performances
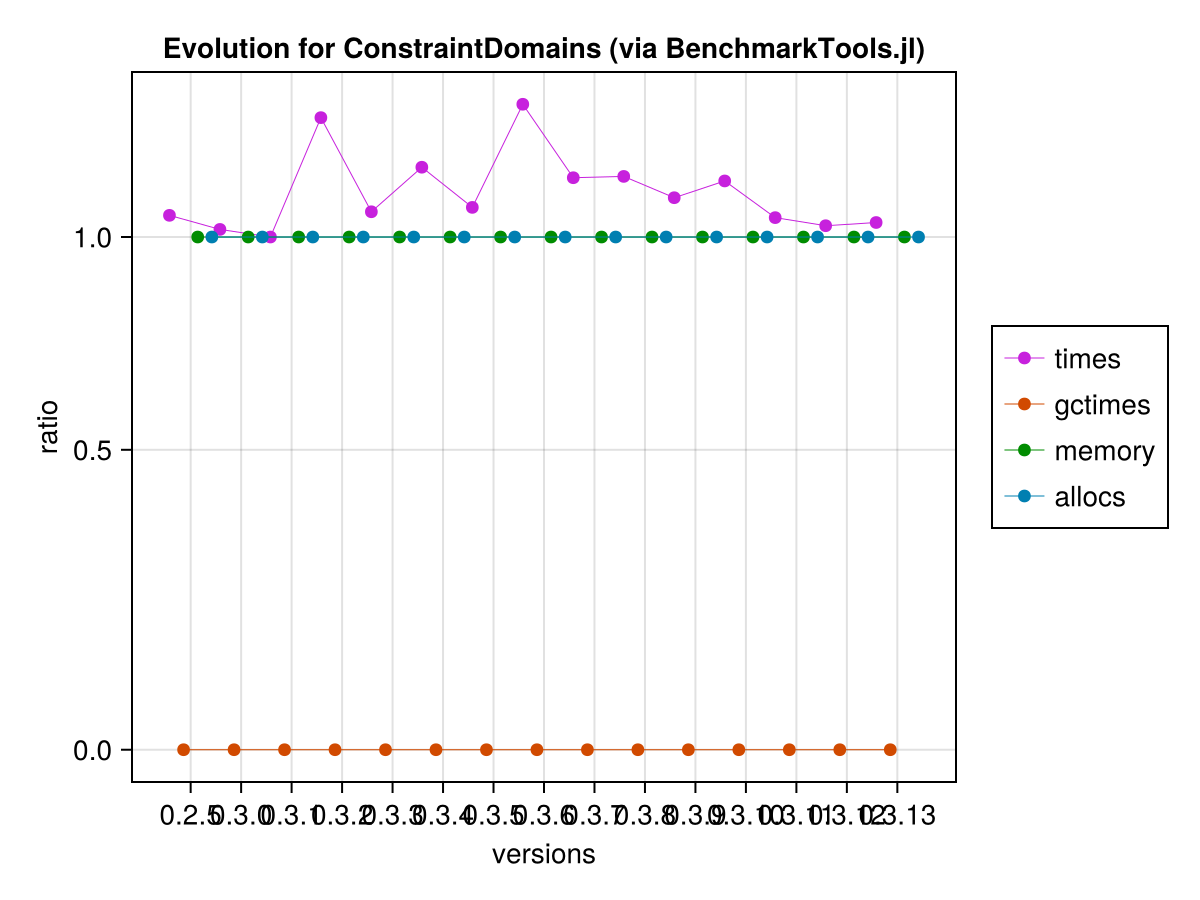
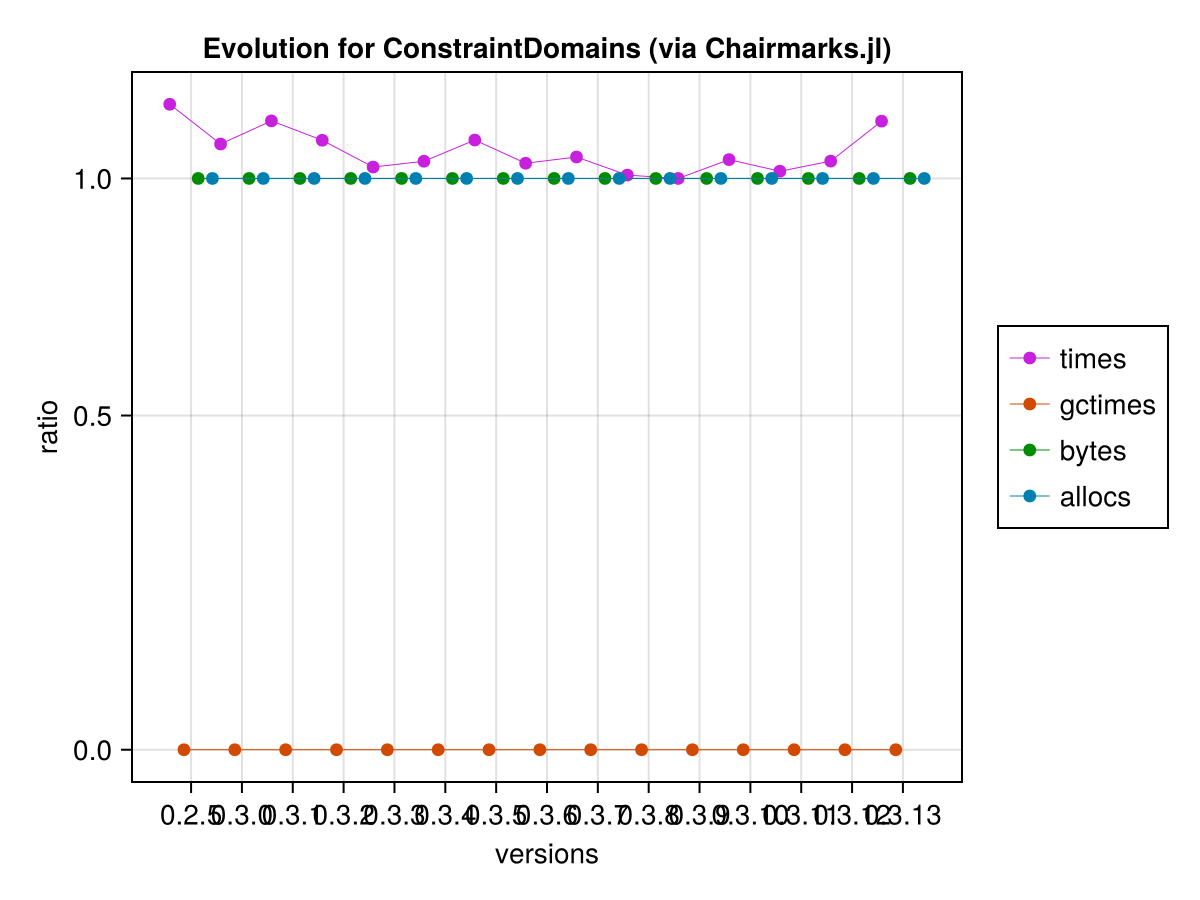
General
Base.convert Function
Base.convert(::Type{Union{Intervals, RangeDomain}}, d::Union{Intervals, RangeDomain})
Extends Base.convert
for domains.
Exploration
ConstraintDomains.Explorer Type
Explorer(concepts, domains, objective = nothing; settings = ExploreSettings(domains))
Create an Explorer object for searching a constraint satisfaction problem space.
Arguments
concepts
: A collection of tuples, each containing a concept function and its associated variable indices.domains
: A collection of domains representing the search space.objective
: An optional objective function for optimization problems.settings
: AnExploreSettings
object to configure the exploration process.
Returns
An Explorer
object ready for exploration.
Example
domains = [domain([1, 2, 3]), domain([4, 5, 6])]
concepts = [(sum, [1, 2])]
objective = x -> x[1] + x[2]
explorer = Explorer(concepts, domains, objective)
ConstraintDomains.ExploreSettings Type
ExploreSettings(
domains;
complete_search_limit = 10^6,
max_samplings = sum(domain_size, domains),
search = :flexible,
solutions_limit = floor(Int, sqrt(max_samplings)),
)
Create settings for the exploration of a search space composed by a collection of domains.
Arguments
domains
: A collection of domains representing the search space.complete_search_limit
: Maximum size of the search space for complete search.max_samplings
: Maximum number of samples to take during partial search.search
: Search strategy (:flexible
,:complete
, or:partial
).solutions_limit
: Maximum number of solutions to store.
Returns
An ExploreSettings
object.
Example
domains = [domain([1, 2, 3]), domain([4, 5, 6])]
settings = ExploreSettings(domains, search = :complete)
ConstraintDomains.explore! Function
explore!(explorer::Explorer)
Perform exploration on the search space defined by the Explorer
object.
This function explores the search space according to the settings specified in the Explorer
object. It updates the Explorer
's state with found solutions and non-solutions.
Arguments
explorer
: AnExplorer
object containing the problem definition and exploration settings.
Returns
Nothing. The Explorer
's state is updated in-place.
Example
explorer = Explorer(concepts, domains)
explore!(explorer)
println("Solutions found: ", length(explorer.state.solutions))
ConstraintDomains.explore Function
explore(domains, concept; settings = ExploreSettings(domains), parameters...)
Explore a search space defined by domains and a concept.
Arguments
domains
: A collection of domains representing the search space.concept
: The concept function defining the constraint.settings
: AnExploreSettings
object to configure the exploration process.parameters
: Additional parameters to pass to the concept function.
Returns
A tuple containing two sets: (solutions, non_solutions).
Example
domains = [domain([1, 2, 3]), domain([4, 5, 6])]
solutions, non_solutions = explore(domains, allunique)
Performances
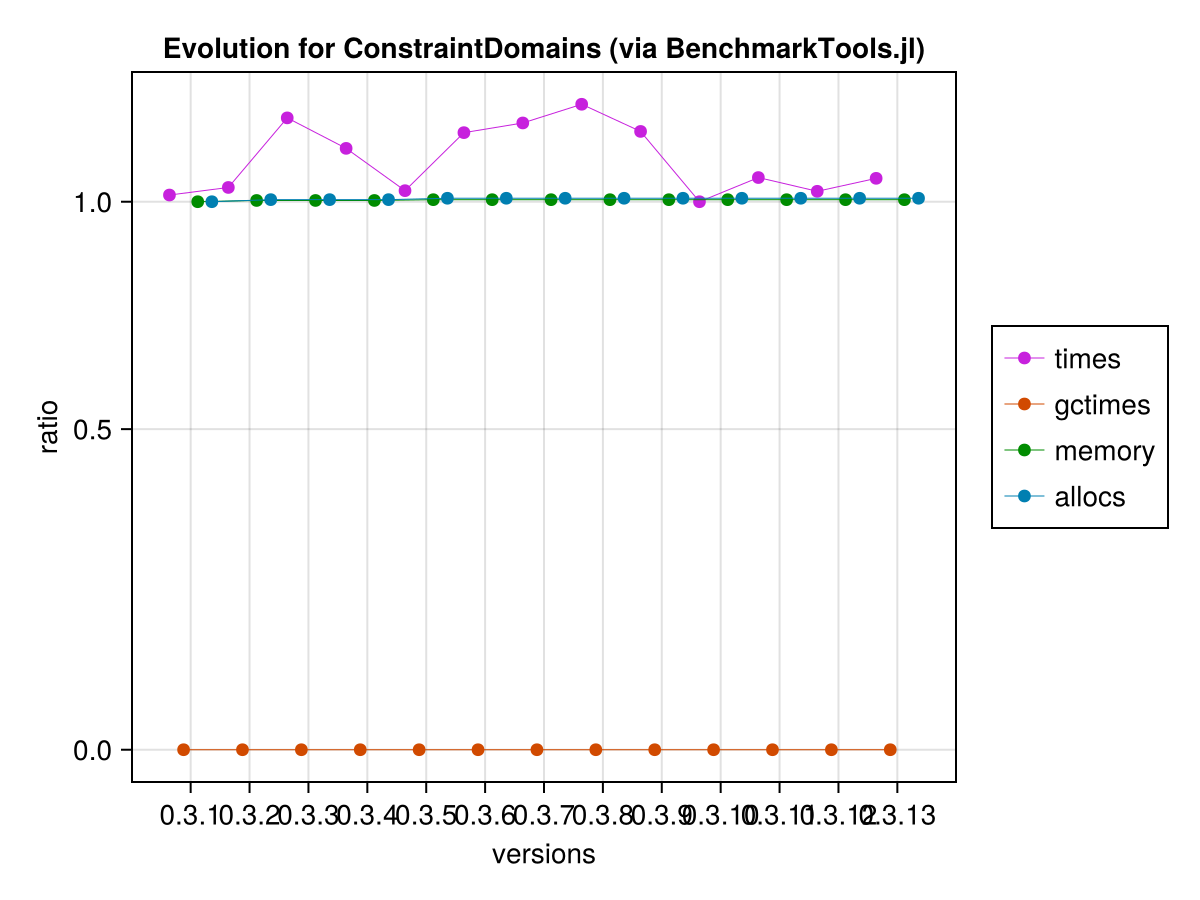
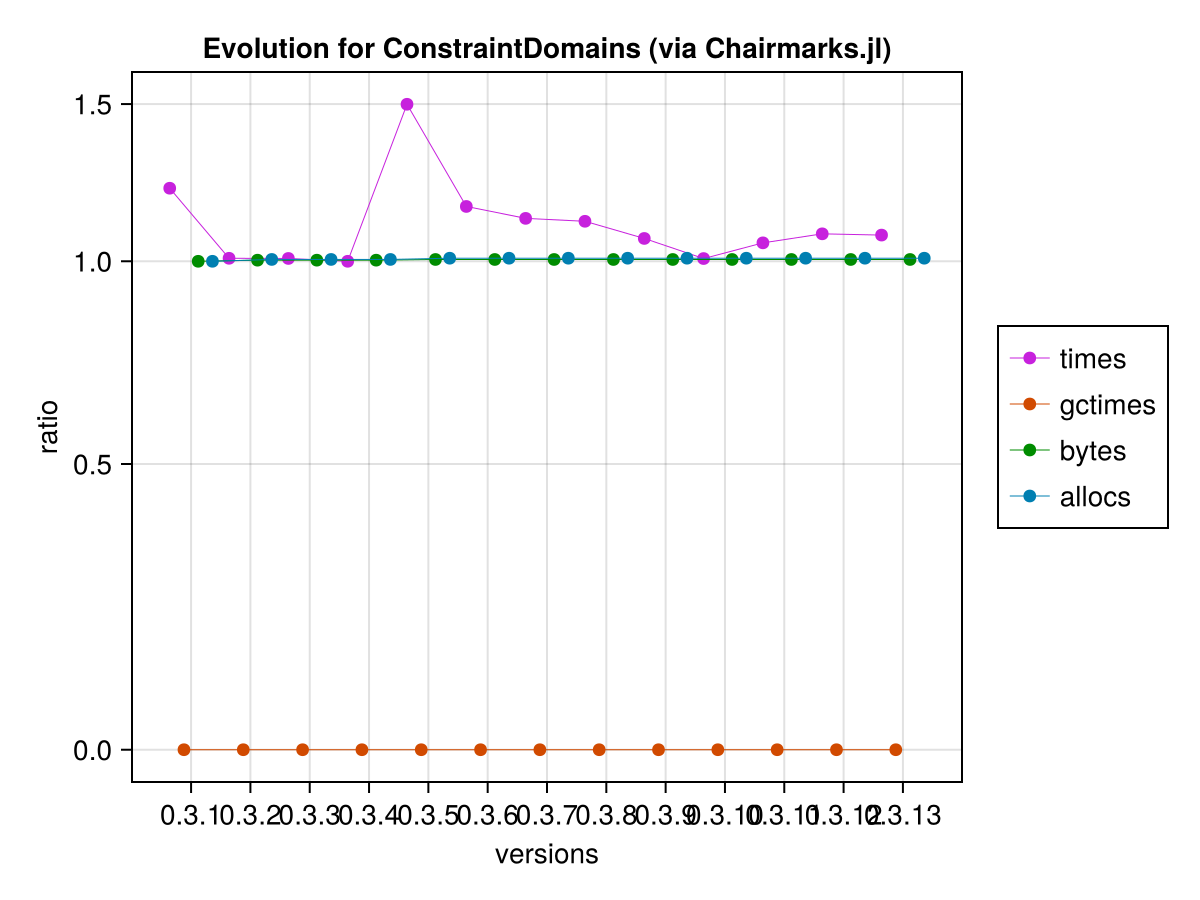
Parameters
ConstraintDomains.BoolParameterDomain Type
BoolParameterDomain <: AbstractDomain
A domain to store boolean values. It is used to generate random parameters.
ConstraintDomains.DimParameterDomain Type
DimParameterDomain <: AbstractDomain
A domain to store dimensions. It is used to generate random parameters.
ConstraintDomains.IdParameterDomain Type
IdParameterDomain <: AbstractDomain
A domain to store ids. It is used to generate random parameters.
ConstraintDomains.FakeAutomaton Type
FakeAutomaton{T} <: ConstraintCommons.AbstractAutomaton
A structure to generate pseudo automaton enough for parameter exploration.
ConstraintCommons.accept Function
accept(a::Union{Automaton, MDD}, w)
Return true
if a
accepts the word w
and false
otherwise.
ConstraintCommons.accept(fa::FakeAutomaton, word)
Implement the accept
methods for FakeAutomaton
.
ConstraintDomains.LanguageParameterDomain Type
LanguageParameterDomain <: AbstractDomain
A domain to store languages. It is used to generate random parameters.
ConstraintDomains.OpParameterDomain Type
OpParameterDomain{T} <: AbstractDomain
A domain to store operators. It is used to generate random parameters.
ConstraintDomains.PairVarsParameterDomain Type
PairVarsParameterDomain{T} <: AbstractDomain
A domain to store values paired with variables. It is used to generate random parameters.
ConstraintDomains.ValParameterDomain Type
ValParameterDomain{T} <: AbstractDomain
A domain to store one value. It is used to generate random parameters.
ConstraintDomains.ValsParameterDomain Type
ValsParameterDomain{T} <: AbstractDomain
A domain to store values. It is used to generate random parameters.
Base.rand Function
Base.rand(::Vector{IntervalsFold})
Extend the Base.rand
function to Vector{IntervalsFold}
.
rand(pf<:PatternFold)
Returns a random value of pf
as if it was unfolded.
Base.rand(::Vector{AbstractVectorFold})
Extend the `Base.rand` function to `Vector{AbstractVectorFold}`.
Base.rand(d::Union{Vector{D},Set{D}, D}) where {D<:AbstractDomain}
Extends Base.rand
to (a collection of) domains.
Base.rand(itv::Intervals)
Base.rand(itv::Intervals, i)
Return a random value from itv
, specifically from the i
th interval if i
is specified.
Base.rand(d::D) where D <: DiscreteDomain
Draw randomly a point in d
.
Base.rand(fa::FakeAutomaton)
Extends Base.rand
. Currently simply returns fa
.
ConstraintDomains.generate_parameters Function
generate_parameters(d<:AbstractDomain, param)
Generates random parameters based on the domain d
and the kind of parameters param
.